How to Use SSTuino II's Onboard RGB LED
Table of Contents
Overview
Ever notice that your SSTuino II has an RGB LED on the board itself? This tutorial will walk you through how to use it!
The RGB LED is physically connected to the Wi-Fi chip (ESP32) and must be controlled through the WiFiNINA interface, which has a special function to set the LED's brightness.
Wiring it up
There is no need to wire anything externally to your SSTuino II. It can even operate completely independently of the Explorer board. The ESP32 Wi-Fi chip is physically wired to the ATmega4809 main microcontroller via SPI pins and communicates solely via SPI.
Code
Basic Tutorial
Open an existing Arduino sketch, or create a new sketch and ensure that you have imported the two necessary libraries for operating with the Wi-Fi chip:
#include <SPI.h>
#include <WiFiNINA.h>
Afterwards, you can use the function below to change the brightness of the RGB LED however you like:
WiFi.setLEDs(255, 255, 255);
The 3 parameters represent the value of red, green and blue respectively. If you have previously used analogWrite()
, this is very similar. The maximum and minimum values are 255 and 0 respectively, with 255 being the LED at maximum brightness and 0 switching off the LED.
If you wish to download the full sample program, you can download it below:
Download the Simple RGB Program
Switching it up (Advanced Tutorial)
Do you want to make it look similar to what the SSTuino II looked like when you first connected it? Well, you can do that!
There are two parts to the program used for the code. At first, the LED will cycle all its colours independently: red, green, followed by blue. Place this code in the setup()
part of your sketch to do exactly that.
WiFi.setLEDs(32, 0, 0);
delay(500);
WiFi.setLEDs(0, 32, 0);
delay(500);
WiFi.setLEDs(0, 0, 32);
delay(500);
Afterwards, you want your LED to slowly fade from one colour to the next. Let's go through the logic: slowly increase the brightness of one colour while decreasing the other one, until the first one reaches maximum brightness while the previous colour reaches 0 (completely turns off). Now, the colour will need to change (if it was originally red to green, it will now need to be green to blue, and then blue to red, and so on and so forth).
Note: notice that we are only using 32 in our example and not the highest possible value, 255. Why is that so? The reason is that 255 is typically way too bright, and we found that 32 was a good amount that could be seen in most lighting conditions.
Firstly, you will need an array to contain the values (0-255) of the 3 colours. An array is a special type of variable that stores multiple other variables. Think of it like a pill box organiser:
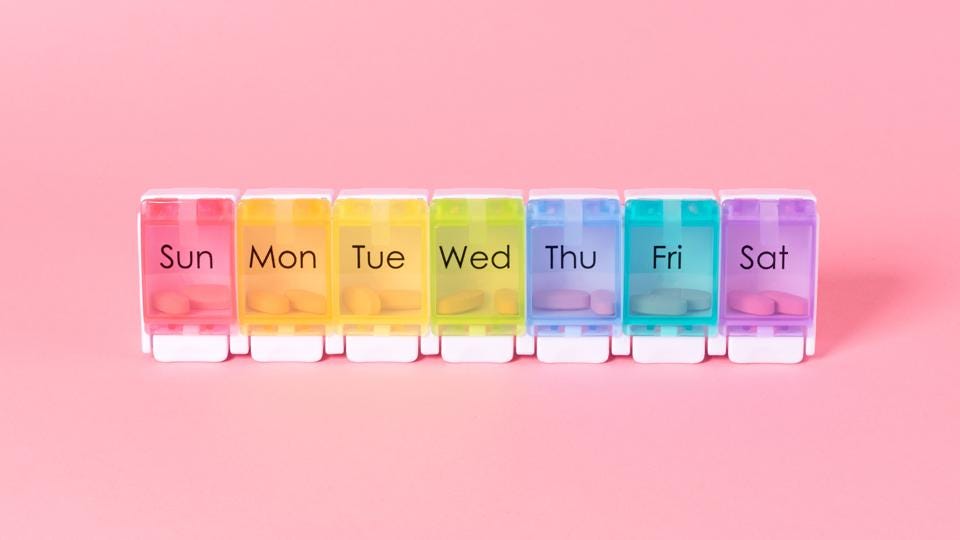
A pill box organiser like the one you see above has 7 slots, one for each day. You can put pills in each box, take them out, or simply leave that slot empty.
For our case, we only need to contain 3 colours (red, green and blue) and hence we will only need an array with 3 "slots". Set all the slots to 0 except the first slot (red), which we will put as 32.
int rgbColour[3];
// Start off with red (set red to 32/255)
rgbColour[0] = 32;
rgbColour[1] = 0;
rgbColour[2] = 0;
Next, you should make a for loop to cycle through all the possible colours (specifically, the colours that are supposed to decrease in brightness). There are only 3 colours in total, so limit the for loop to stop at 3.
Next, the program will need to select a colour that should be increasing. For example, if the current decreasing colour is red, the increasing colour should be green, and then blue, and then back to red. For this task, we wrote a one-line ternary operator: int incColour = decColour == 2 ? 0 : decColour + 1;
. This one line does the same thing as the 6 lines of code below:
int incColour;
if (decColour == 2) {
incColour = 0;
} else {
incColour = decColour + 1;
}
Finally, you can start to write the inner loop for actually fading the colours, by decrementing the value of 1 colour and incrementing the value of another colour.
for (int i = 0; i < 32; i += 1)
{
rgbColour[decColour] -= 1;
rgbColour[incColour] += 1;
WiFi.setLEDs(rgbColour[0], rgbColour[1], rgbColour[2]);
delay(60);
}
At last, your program loop()
should look something like this:
void loop()
{
// This code increases the intensity of one colour, while
// fading the other colour, and cycles to another colour
// once the initial one is finished
int rgbColour[3];
// Start off with red (set red to 32/255)
rgbColour[0] = 32;
rgbColour[1] = 0;
rgbColour[2] = 0;
// Choose the colours to increment and decrement.
for (int decColour = 0; decColour < 3; decColour += 1)
{
int incColour = decColour == 2 ? 0 : decColour + 1;
// cross-fade the two colours.
for (int i = 0; i < 32; i += 1)
{
rgbColour[decColour] -= 1;
rgbColour[incColour] += 1;
WiFi.setLEDs(rgbColour[0], rgbColour[1], rgbColour[2]);
delay(60);
}
}
}
Complete code
/*
RGB LED program for the SSTuino II
Flashes all the LEDs inside the RGB LED
one by one, and then cross fades two colours
Written by Pan Ziyue for FourierIndustries
Licensed under the Creative Commons 0 (CC0) License and
released to public domain
<http://creativecommons.org/publicdomain/zero/1.0/>
*/
#include <SPI.h>
#include <WiFiNINA.h>
void setup()
{
// Perform a single 1/8th strength cycle through on
// pure colours, max value possible is 255
WiFi.setLEDs(32, 0, 0);
delay(500);
WiFi.setLEDs(0, 32, 0);
delay(500);
WiFi.setLEDs(0, 0, 32);
delay(500);
}
void loop()
{
// This code increases the intensity of one colour, while
// fading the other colour, and cycles to another colour
// once the initial one is finished
int rgbColour[3];
// Start off with red (set red to 32/255)
rgbColour[0] = 32;
rgbColour[1] = 0;
rgbColour[2] = 0;
// Choose the colours to increment and decrement.
for (int decColour = 0; decColour < 3; decColour += 1)
{
int incColour = decColour == 2 ? 0 : decColour + 1;
// cross-fade the two colours.
for (int i = 0; i < 32; i += 1)
{
rgbColour[decColour] -= 1;
rgbColour[incColour] += 1;
WiFi.setLEDs(rgbColour[0], rgbColour[1], rgbColour[2]);
delay(60);
}
}
}
Resources and Going Further
If you wish to explore more about the Wi-Fi chip's specific features that you can invoke in the WiFiNINA library, you can check out some of the resources below: