Utilising Smart LED Strips with SSTuino II
Table of Contents
Overview
Have you ever seen products like Razer's Chroma and thought to yourself: wouldn't it be great if I could make one myself? Well, now you can!
Most smart LED strips on the market use a special kind of RGB LED called a "WS2812B" LED. Sometimes, they are marketed online as "NeoPixels" by Adafruit, or simply "individually addressable LEDs" by other manufacturers. Most of these products are compatible with each other and the terms above are interchangeable, to a certain degree. For convenience's sake, we will call them "smart LEDs".
The WS2812B is a tiny computer chip that sits inside the LED package, allowing you to control the smart LED's brightness without having to have 3 digital pins doing analogWrite()
. Furthermore, you can daisy chain the LEDs to each other to individually control other LEDs down the LED strip.
On the left image below, you can see an ordinary RGB LED. On the right side, you can see this smart RGB LED that has the WS2812B chip inside the LED package.
The major advantage of using smart LEDs is that you only need 1 single wire to control a lot of LEDs at once, which is especially useful if you need to control an entire array or strip of LEDs.
Wiring it up
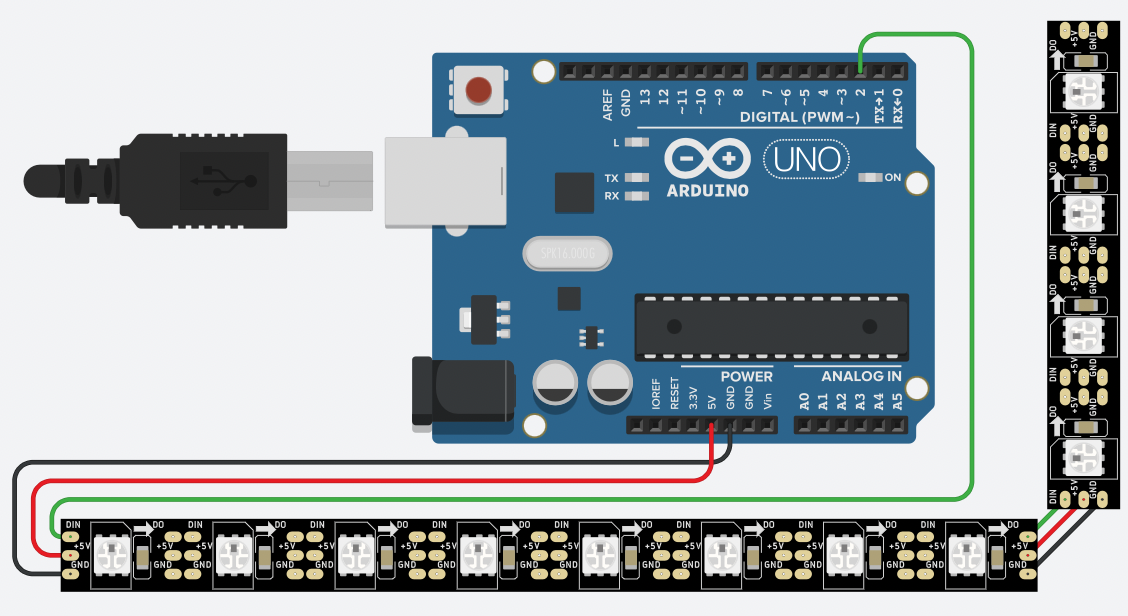
The wiring diagram for using a smart LED is incredibly simple. Simply connect the 5V terminal of the strip to the corresponding 5V on your SSTuino, GND to GND, and DIN (data in) to a data pin of your choice.
Warning: smart LED strips can draw a lot of current! Your SSTuino can usually power up to 40 of these smart LEDs before requiring an external 5V power source. If you need to build a project with extremely long smart LED strips (>100), multiple power sources might need to be provided along the length of the LED strip to prevent dimming and power loss.
Code
Software Setup
You will need to install an external library to your Arduino IDE, called "Adafruit NeoPixel". Simply open the Library Manager under the Tools top bar menu and search for "neopixel".
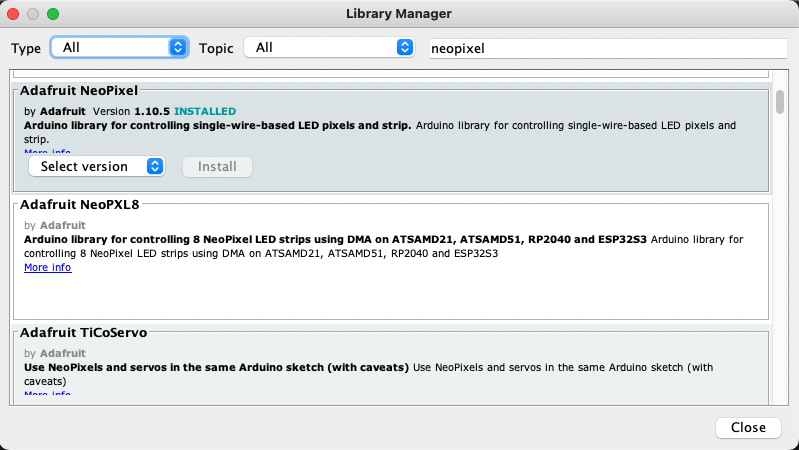
Example code
This code below will import the Adafruit NeoPixel library, and set the maximum number of LEDs in your LED strip to 12 bulbs in #define NUMPIXELS 12
(remember, the maximum your SSTuino can go is 40 bulbs, any more and the LED strip will require external power, or limit its own brightness).
In the setup()
section, simply initialise the library.
Afterwards, you will create a custom function called setColor()
which picks a random value between 0 and 255 (completely off and completely on) for each of the colours: red, green and blue.
Within loop()
, you will call setColor()
once to set the colour values inside the variables redColor
, greenColor
and blueColor
, but not actually show the colours on the LED strip yet. Afterwards, create a for loop that goes through each of the LEDs ("pixels", as termed by Adafruit) and actually displays the colour.
#include <Adafruit_NeoPixel.h>
#define PIN 2 // input pin Neopixel is attached to
#define NUMPIXELS 12 // number of neopixels in strip
Adafruit_NeoPixel pixels = Adafruit_NeoPixel(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800);
int delayval = 100; // timing delay in milliseconds
int redColor = 0;
int greenColor = 0;
int blueColor = 0;
void setup() {
// Initialise the NeoPixel library.
pixels.begin();
}
void loop() {
setColor();
for (int i=0; i < NUMPIXELS; i++) {
// pixels.Color takes RGB values, from 0,0,0 up to 255,255,255
pixels.setPixelColor(i, pixels.Color(redColor, greenColor, blueColor));
// This sends the updated pixel color to the hardware.
pixels.show();
// Delay for a period of time (in milliseconds).
delay(delayval);
}
}
// setColor()
// picks random values to set for RGB
void setColor(){
redColor = random(0, 255);
greenColor = random(0,255);
blueColor = random(0, 255);
}
Troubleshooting
- My smart LED bulbs are flickering/not giving the correct output!: This is most likely an issue with your LED strips. As smart LED strips require the signal to be propagated from one LED to the next like a game of Telephone, if one of the LEDs is faulty, it will result in a corrupted signal for the next LED. You will need to replace your smart LED strip.
Resources and Going Further
Smart LEDs like the WS2812B are commonly used in many projects requiring fancy, individually addressable LED lighting solutions. The Adafruit NeoPixel library we showed in this example is the easiest way to get started, but if you desire higher levels of functionality, you should use FastLED, a more powerful and advanced library for use with microcontrollers.